前言
Core Animation,中文翻译为核心动画,它是一组非常强大的动画处理API,使用它能做出非常炫丽高效的动画效果。
Core Animation可以用在Mac OS X和iOS平台。
Core Animation的动画执行过程都是在后台操作的,不会阻塞主线程。
要注意的是,Core Animation是直接作用在`CALayer上的,并非UIView。
CALayer的属性
既然Core Animation是作用在CALayer上的,那么我们就要先了解下CALayer的一些常用属性:
1 | /* The bounds of the layer. Defaults to CGRectZero. Animatable. */ |
- bounds:大小
- position: 位置(默认指中点,具体由anchorPoint决定)
- anchorPoint:锚点(x,y的范围都是0-1),决定了position
- transform: 形变属性
- backgroundColor: 背景颜色(CGColorRef类型)
- opacity: 透明度
position和anchorPoint
position用来设置CALayer在父层中的位置
以父层的左上角为原点(0, 0)。
anchorPoint称为“定位点”、“锚点”,决定着CALayer身上的哪个点会在position属性所指的位置。以自己的左上角为原点(0, 0),它的x、y取值范围都是0~1,默认值为中心点(0.5, 0.5)
关于position和anchorPoint可参考iOS 理解position与anchorPoint
隐式动画
每一个UIView内部都默认关联着一个CALayer,我们可用称这个Layer为Root Layer(根层),所有的非Root Layer,也就是手动创建的CALayer对象,都存在着隐式动画.
当对非Root Layer的部分属性进行修改时,默认会自动产生一些动画效果,而这些属性称为Animatable Properties(可动画属性)。
可以通过事务关闭隐式动画:1
2
3
4
5
6
7
8[CATransaction begin];
/**关闭隐式动画**/
[CATransaction setDisableActions:YES];
layer.position = CGPointMake(100, 100);
[CATransaction commit];
Core Animation结构
Core Animation结构如图:
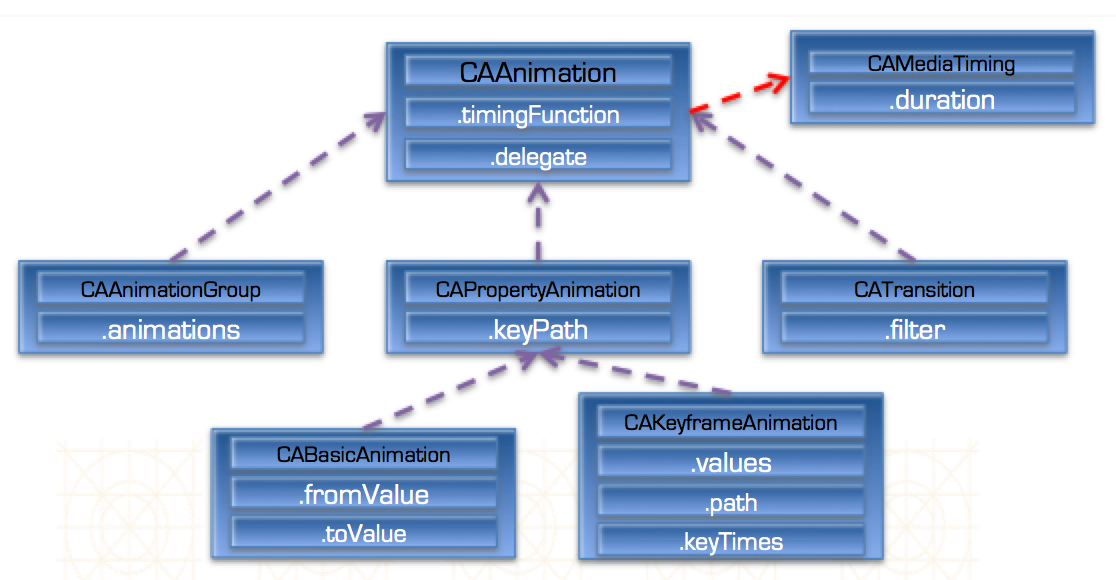
其中灰色虚线表示继承关系,红色表示遵守协议。
核心动画中所有类都遵守CAMediaTiming协议。
CAAnaimation是个抽象类,不具备动画效果,必须用它的子类才有动画效果。
CAAnimationGroup和CATransition才有动画效果,CAAnimationGroup是个动画组,可以同时进行缩放,旋转(同时进行多个动画)。
CATransition是转场动画,界面之间跳转(切换)都可以用转场动画。
CAPropertyAnimation也是个抽象类,本身不具备动画效果,只有子类才有。
CABasicAnimation基本动画,做一些简单效果。
CAKeyframeAnimation帧动画,做一些连续的流畅的动画。
CAAnimation——简介
CAAnimation是所有动画对象的父类,负责控制动画的持续时间和速度,是个抽象类,不能直接使用,应该使用它具体的子类.
CAAnimation属性
duration: 动画的持续时间
repeatCount : 重复次数,无限循环可以设置HUGE_VALF或者MAXFLOAT
repeatDuration : 重复时间
removedOnCompletion :默认为YES,代表动画执行完毕后就从图层上移除,图形会恢复到动画执行前的状态。如果想让图层保持显示动画执行后的状态,那就设置为NO,不过还要设置fillMode为kCAFillModeForwards
fillMode : 决定当前对象在非active时间段的行为。比如动画开始之前或者动画结束之
beginTime : 可以用来设置动画延迟执行时间,若想延迟2s,就设置为CACurrentMediaTime()+2,CACurrentMediaTime()为图层的当前时间
timingFunction : 速度控制函数,控制动画运行的节奏
- delegate : 动画代理
fillMode枚举
kCAFillModeRemoved: 这个是默认值,也就是说当动画开始前和动画结束后,动画对layer都没有影响,动画结束后,layer会恢复到之前的状态
kCAFillModeForwards: 当动画结束后,layer会一直保持着动画最后的状态
kCAFillModeBackwards: 在动画开始前,只需要将动画加入了一个layer,layer便立即进入动画的初始状态并等待动画开始。
kCAFillModeBoth: 这个其实就是上面两个的合成.动画加入后开始之前,layer便处于动画初始状态,动画结束后layer保持动画最后的状态
CAMediaTimingFunction枚举
kCAMediaTimingFunctionLinear(线性):匀速,给你一个相对静态的感觉
kCAMediaTimingFunctionEaseIn(渐进):动画缓慢进入,然后加速离开
kCAMediaTimingFunctionEaseOut(渐出):动画全速进入,然后减速的到达目的地
kCAMediaTimingFunctionEaseInEaseOut(渐进渐出):动画缓慢的进入,中间加速,然后减速的到达目的地。这个是默认的动画行为。
CABasicAnimation——基本动画
基本动画,执行过程随着动画的进行,在长度为duration的持续时间内,keyPath相应属性的值从fromValue渐渐地变为toValue。
示例代码:
1 | - (void)lyz_basicAnimation{ |
如果fillMode = kCAFillModeForwards同时removedOnComletion = NO,那么在动画执行完毕后,图层会保持显示动画执行后的状态。但在实质上,图层的属性值还是动画执行前的初始值,并没有真正被改变。
CAKeyframeAnimation——关键帧动画
关键帧动画,也是CAPropertyAnimation的子类,与CABasicAnimation的区别是:
CABasicAnimation只能从一个数值(fromValue)变到另一个数值(toValue),而CAKeyframeAnimation会使用一个NSArray保存这些数值
CABasicAnimation可看做是只有2个关键帧的CAKeyframeAnimation
示例代码:
1 | - (void)lyz_keyframeAnimatin{ |
CAAnimationGroup——动画组
动画组,是CAAnimation的子类,可以保存一组动画对象,将CAAnimationGroup对象加入层后,组中所有动画对象可以同时并发运行。
默认情况下,一组动画对象是同时运行的,也可以通过设置动画对象的beginTime属性来更改动画的开始时间。
示例代码:
1 | - (void)lyz_groupAnimation{ |
CATransition——转场动画
它是CAAnimation的子类,用于做过渡动画或者转场动画,能够为层提供移出屏幕和移入屏幕的动画效果。 其中最主要的就是 type 和 subType 这两个属性。
1 | @property(copy) NSString *type |
Type: 动画的类型
1 | /* Common transition types. */ |
SubType: 动画类型的方向1
2
3
4
5
6
7
8
9
10/* Common transition subtypes. */
CA_EXTERN NSString * const kCATransitionFromRight
CA_AVAILABLE_STARTING (10.5, 2.0, 9.0, 2.0);
CA_EXTERN NSString * const kCATransitionFromLeft
CA_AVAILABLE_STARTING (10.5, 2.0, 9.0, 2.0);
CA_EXTERN NSString * const kCATransitionFromTop
CA_AVAILABLE_STARTING (10.5, 2.0, 9.0, 2.0);
CA_EXTERN NSString * const kCATransitionFromBottom
CA_AVAILABLE_STARTING (10.5, 2.0, 9.0, 2.0);
示例代码:
1 | - (void)lyz_transitionAnimation{ |
总结
以上就是一些对Core Animation的理解和认识,如果需要Demo可以到这里下载。写的不好的地方请大家批评指正,谢谢。